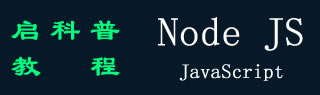
- Node.js 菜鸟教程
- Node.js - 教程
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 首次申请
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 活动
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 流程
- Node.js - 扩展应用程序
- Node.js - 包装
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲器
- Node.js - Streams
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL创建数据库
- Node.js - MySQL创建表
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where 子句
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - utility 模块
- Node.js - Web 模块
NodeJS - url.port 属性
NodeJS url.port 属性是 URL 模块中 URL 类的内置应用程序编程接口。
此属性设置并获取所提供 URL 的端口部分。端口段中的值可以是数字或字符串,其中包含 0 到 65535(含)之间的数字。将该值设置为给定协议的 URL 对象的默认端口将导致端口值变为空字符串 (“)。
端口值可以是空字符串,在这种情况下,端口取决于协议/方案。以下是协议和端口值的列表
协议 | 端口 |
---|---|
“ftp” | 21 |
“file” | |
“http” | 80 |
“https” | 443 |
“ws” | 80 |
“wss” | 443 |
将值分配给端口段后,将首先使用 toString() 方法将该值转换为字符串。如果字符串无效但以数字开头,则前导数字将分配给端口值。
语法
以下是 URL 类的 NodeJS 端口属性的语法
URL.port
参数
此属性不接受任何参数。
返回值
此属性获取并设置所提供 URL 的端口部分。
例如果我们将完整的 URL 分配给 NodeJS url.port 属性,它将获取给定 URL 的端口部分。
在下面的以下示例中,我们尝试从输入 URL 获取端口段中的值。
const http = require('url');
const myURL = new URL('https://www.qikepu.com:2692');
console.log("Port portion of the URL is: " + myURL.port);
输出
执行上述程序后,port 属性从提供的 URL 中获取端口段。
例
如果将端口值设置为 URL 对象的指定协议的默认端口,则端口值将变为空字符串 (' ')。
在以下示例中,我们在 URL 的端口段中设置 https 和 ftp 协议的默认端口值。
const http = require('url');
const myURL1 = new URL('https://www.qikepu.com:443');
console.log("Port portion of the URL is: " + myURL1.port);
const myURL2 = new URL('ftp://www.qikepu.com:21');
console.log("Port portion of the URL is: " + myURL2.port);
输出
正如我们在下面的输出中看到的,port 属性将 https 和 ftp 协议的默认端口值设置为空字符串。
Port portion of the URL is: ''
例
URL 中端口段的值只能包含一个数字或一个包含 0 到 65535 之间数字的字符串。
在下面的程序中,我们尝试为输入 URL 的端口段设置一个数字 (4523)。
const http = require('url');
const myURL = new URL('https://www.qikepu.com');
console.log("The URL: " + myURL);
console.log("Port portion of the URL is: " + myURL.port);
myURL.port = 4523;
console.log("Modified the value in port portion to: " + myURL.port);
console.log(myURL.href);
输出
正如我们在下面的输出中看到的,port 属性允许将 4523 设置为端口段。
Port portion of the URL is:
Modified the value in port portion to: 4523
https://www.qikepu.com:4523/
例
如果我们尝试在端口段中包含完全无效的端口字符串,port 属性将忽略它们。
在以下示例中,我们尝试将包含字母的字符串设置为 URL 的端口段。
const http = require('url');
const myURL = new URL('https://www.qikepu.com:5678');
console.log("The URL: " + myURL);
console.log("Port portion of the URL is: " + myURL.port);
myURL.port = "abcdefg";
console.log("Trying to set the port value as: ", "abcdefg");
console.log("After modifying: " + myURL.href);
输出
正如我们在下面的输出中看到的,port 属性忽略了无效的端口字符串。
Port portion of the URL is: 5678
Trying to set the port value as: abcdefg
After modifying: https://www.qikepu.com:5678/
例
如果我们尝试将包含字母数字值的字符串设置为端口段,port 属性会将前导数字视为端口号。
在下面的示例中,我们尝试为端口段设置一个字母数字值。
const http = require('url');
const myURL = new URL('https://www.qikepu.com:5678');
console.log("The URL: " + myURL);
console.log("Port portion of the URL is: " + myURL.port);
myURL.port = "2345vniu";
console.log("Trying to set the port value as: ", "2345vniu");
console.log("After modifying: " + myURL.href);
输出
如果我们编译并运行上述程序,port 属性会将前导数字视为端口值。
Port portion of the URL is: 5678
Trying to set the port value as: 2345vniu
After modifying: https://www.qikepu.com:2345/
例
如果将包含非整数(如浮点数)的数字或字符串设置为端口段,则端口属性将截断并仅将小数点前的数字视为端口值。
在以下示例中,我们尝试为端口段设置非整数值。
const http = require('url');
const myURL = new URL('https://www.qikepu.com:999');
console.log("The URL: " + myURL);
console.log("Port portion of the URL is: " + myURL.port);
myURL.port = "123.456";
console.log("Trying to set the port value as: ", "123.456");
console.log("After modifying: " + myURL.href);
输出
正如我们在下面的输出中看到的,port 属性被截断,只考虑小数点前的数字。
Port portion of the URL is: 999
Trying to set the port value as: 123.456
After modifying: https://www.qikepu.com:123/
例
如果我们尝试为端口段设置超出范围的数字,port 属性将忽略它。
在以下示例中,我们尝试将包含超出范围的数字的字符串设置为 URL 的端口段。
const http = require('url');
const myURL = new URL('https://www.qikepu.com:6345');
console.log("The URL: " + myURL);
console.log("Port portion of the URL is: " + myURL.port);
myURL.port = "6553556";
console.log("Trying to set the port value as: ", "6553556");
console.log("After modifying: " + myURL.href);
输出
执行上述程序后,我们尝试设置的值被忽略,因为它超出了可接受的范围。
Port portion of the URL is: 6345
Trying to set the port value as: 6553556
After modifying: https://www.qikepu.com:6345/