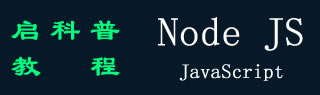
- Node.js教程
- Node.js - 教程
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 首次申请
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 活动
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 流程
- Node.js - 扩展应用程序
- Node.js - 包装
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲器
- Node.js - Streams
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL创建数据库
- Node.js - MySQL创建表
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where 子句
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - utility 模块
- Node.js - Web 模块
Node.js - os.freemem() 方法
Node.js 的 os 模块提供了一堆与操作系统相关的实用方法和属性。在本章中,我们将通过适当的示例学习 os 模块的 os.freemem() 方法。
Node.js os.freemem() 方法将以整数形式返回当前计算机 RAM 中剩余的可用内存量(以字节为单位)。此值将始终小于 totalmem,后者返回总系统内存(以字节为单位)。返回的值可用于确定进程是否需要更多内存,或者是否应由于缺少资源而缩减其操作。
语法
以下是Node.js os.freemem()方法的语法 -
os.freemem()
参数
此方法不接受任何参数。
返回值
此方法将以整数值的形式返回可用系统内存量(以字节为单位)。
以下是转换图表 -
- 1024 字节 = 1KB(千字节)
- 1024 KB = 1 MB(兆字节)
- 1024 MB = 1 GB(千兆字节)
- 1024 GB = 1 TB (太字节)
- 1024 TB = 1 PB(PB 级)
在以下示例中,我们尝试使用 os.freemem() 方法打印当前系统的可用空间。
const os = require('os');
console.log(os.freemem());
输出
130521513984
注意 - 为了获得准确的结果,最好在本地执行上述代码。
如果我们编译并运行上述程序,os.freemem() 将返回当前计算机的可用系统内存(以字节为单位)。
9211826176
例
在下面的示例中,我们尝试将可用系统内存量从字节转换为 KB(千字节)、MB(兆字节)和 GB(千兆字节)。
const os = require('os');
var mem_in_bytes = os.freemem();
var mem_in_kb = Math.floor(os.freemem()/(1024));
var mem_in_mb = Math.floor(os.freemem()/(1024*1024));
var mem_in_gb = Math.floor(os.freemem()/(1024*1024*1024));
console.log("The amount of free current system memory(in bytes): " + mem_in_bytes);
console.log("The amount of free current system memory(in KB): " + mem_in_kb);
console.log("The amount of free current system memory(in MB): " + mem_in_mb);
console.log("The amount of free current system memory(in GB): " + mem_in_gb);
输出
The amount of free current system memory(in bytes): 130537541632
The amount of free current system memory(in KB): 127478068
The amount of free current system memory(in MB): 124490
The amount of free current system memory(in GB): 121
The amount of free current system memory(in KB): 127478068
The amount of free current system memory(in MB): 124490
The amount of free current system memory(in GB): 121
注意 - 为了获得准确的结果,最好在本地执行上述代码。
如果我们编译并运行上述程序,我们将以字节、千字节 (KB)、兆字节 (MB) 和千兆字节 (GB) 为单位打印可用系统内存。
The amount of free current system memory(in bytes): 9202073600
The amount of free current system memory(in KB): 8986400
The amount of free current system memory(in MB): 8775
The amount of free current system memory(in GB): 8
The amount of free current system memory(in KB): 8986400
The amount of free current system memory(in MB): 8775
The amount of free current system memory(in GB): 8