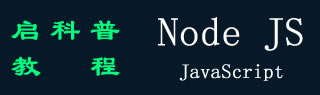
- Node.js 菜鸟教程
- Node.js - 教程
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 首次申请
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 活动
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 流程
- Node.js - 扩展应用程序
- Node.js - 包装
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲器
- Node.js - Streams
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL创建数据库
- Node.js - MySQL创建表
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where 子句
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - utility 模块
- Node.js - Web 模块
NodeJS - console.dir() 方法
Node.js console.dir() 方法是 Console 类的内置方法。
Node.js 的 console.dir() 方法返回特定对象的属性。此方法用于在终端窗口中显示特定对象属性的交互式列表。调用时,它会打印对象属性和值的分层表示形式,使用户能够快速检查它们,而无需手动迭代每个属性或值。
这在调试时特别有用。这是因为与在控制台日志中输入单个属性及其关联值相比,查看对象更容易。
假设一个男孩是一个物体,他有一些属性,如名字、年龄、学校、标记等。为了返回男孩的属性,我们使用 node.js 的 console.dir() 方法。为了更好地理解,让我们研究一下 console.dir() 方法的语法和用法。
语法
以下是Node.js console.dir()方法的语法 -
console.dir(object[, options])
参数
此方法只接受两个参数。下面将对此进行描述。
- object − 此参数指定必须返回的对象。
以下是可选参数,让我们来看看它们。
- showHidden - 这是一个布尔值。如果为 true,则将显示对象的 non-enumerable 和 symbol 属性。
- depth - 这是一个数字。这将让我们打印指定对象的级别。默认情况下,深度为 2。如果我们想获取对象的所有级别,则传递 null。
- colors - 这是一个布尔值。如果我们传递 true,则属性值将以 ANSI 颜色代码显示。我们可以自定义颜色。默认情况下,该值为 false(无 ANSI 颜色)
返回值
此方法返回指定对象的属性。
例Node.js console.dir() 方法的 node.js 将只接受一个对象参数。
在下面的示例中,我们将创建一个具有可枚举属性的对象。为了返回对象属性,我们调用Node.js console.dir() 方法,并将对象名称作为参数传递。
var object = {
Name : "Nik",
Age : 31,
Eyes :"black",
height : 5.2,
weight : 70
}
console.dir(object);
输出
正如我们在下面的输出中看到的,当我们通过将对象名称作为参数传递来调用 console.dir() 方法时,将列出对象的可枚举属性。
例
在下面的示例中,我们正在创建一个 4 级对象。为了返回对象属性,我们调用 console.dir() 方法,并将对象名称作为参数传递。
注意 - 默认情况下,console.dir() 方法最多只能达到 2 个级别。
var object = { // level 0 start
firstname : "James",
lastname : "Anderson",
Initial : function() {
return this.firstname[0] + this.lastname[0];
},
attrributes: { // level 1 start
age : 34,
school : "High school",
hobbies : { // level 2 start
favourite : { // level 3 start
sport : "football",
book : "Nothing"
},
RecentlyPlayed : {
game : "cricket",
videogame : "GTA5"
} // level 3 ends
} // level 2 ends
} // level 1 ends
} // level 0 ends
console.dir(object);
输出
正如我们在下面的输出中看到的,我们得到了对象的属性作为最多 2 个级别的输出。因此,要获取对象的所有属性,我们需要使用 depth 参数。要了解 depth 参数的用法,请查看下一个示例。
firstname: 'James',
lastname: 'Anderson',
Initial: [Function: Initial],
attrributes: {
age: 34,
school: 'High school',
hobbies: { favourite: [Object], RecentlyPlayed: [Object] }
}
}
例
node.js 的 console.dir() 方法也将接受一些额外的参数,如 showHidden、depth 和 colors,我们在示例中使用了 depth 可选参数。
在下面的示例中,
- 我们正在创建一个 4 级对象。
- 为了返回对象属性,我们调用 console.dir() 方法,并将对象名称作为参数传递。
- 默认情况下,它只返回对象的属性,最多返回 2 个级别。
- 我们尝试使用 depth 参数来获取对象的所有级别的属性。
var object = { // level 0 start
firstname : "James",
lastname : "Anderson",
Initial : function() {
return this.firstname[0] + this.lastname[0];
},
attrributes: { // level 1 start
age : 34,
school : "High school",
hobbies : { // level 2 start
favourite : { // level 3 start
sport : "football",
book : "Nothing"
},
RecentlyPlayed : {
game : "cricket",
videogame : "GTA5"
} // level 3 ends
} // level 2 ends
} // level 1 ends
} // level 0 ends
console.dir(object, {depth: null, showHidden: true});
输出
正如我们在下面的输出中看到的,对象的所有级别的属性都被打印出来了。我们通过将深度声明为 null 来做到这一点。
firstname: 'James',
lastname: 'Anderson',
Initial: [Function: Initial] {
[length]: 0,
[name]: 'Initial',
[arguments]: null,
[caller]: null,
[prototype]: Initial { [constructor]: [Circular] }
},
attrributes: {
age: 34,
school: 'High school',
hobbies: {
favourite: { sport: 'football', book: 'Nothing' },
RecentlyPlayed: { game: 'cricket', videogame: 'GTA5' }
}
}
}
例
node.js 的 console.dir() 方法也将接受一些额外的参数,如 showHidden、depth 和 colors,我们在示例中使用了 showHidden 可选参数。
在下面的示例中,
- 我们正在创建一个 4 级对象。
- 为了返回对象属性,我们调用 console.dir() 方法,并将对象名称作为参数传递。
- 我们将 showHidden 参数作为 true 传递,它将显示隐藏对象的不可枚举和符号属性。
var object = { // level 0 start
firstname : "James",
lastname : "Anderson",
Initial : function() {
return this.firstname[0] + this.lastname[0];
},
attrributes: { // level 1 start
age : 34,
school : "High school",
hobbies : { // level 2 start
favourite : { // level 3 start
sport : "football",
book : "Nothing"
},
RecentlyPlayed : {
game : "cricket",
videogame : "GTA5"
} // level 3 ends
} // level 2 ends
} // level 1 ends
} // level 0 ends
console.dir(object, {depth: null, showHidden: true});
输出
正如我们在输出中看到的,隐藏的属性与对象的属性一起显示在输出中。
firstname: 'James',
lastname: 'Anderson',
Initial: <ref *1> [Function: Initial] {
[length]: 0,
[name]: 'Initial',
[arguments]: null,
[caller]: null,
[prototype]: { [constructor]: [Circular *1] }
},
attrributes: {
age: 34,
school: 'High school',
hobbies: {
favourite: { sport: 'football', book: 'Nothing' },
RecentlyPlayed: { game: 'cricket', videogame: 'GTA5' }
}
}
}
例
node.js 的 console.dir() 方法也将接受一些额外的参数,如 showHidden、depth 和 colors,我们在示例中使用 colors 作为可选参数。
在下面的示例中,
- 我们正在创建一个 4 级对象。
- 为了返回对象属性,我们调用 console.dir() 方法,并将对象名称作为参数传递。
- 我们通过将 colors 参数作为 true 传递来更改输出的颜色(使用 ANSI 颜色代码)。默认情况下,该值为 false(无颜色)。
var object = { // level 0 start
firstname : "James",
lastname : "Anderson",
Initial : function() {
return this.firstname[0] + this.lastname[0];
},
attrributes: { // level 1 start
age : 34,
school : "High school",
hobbies : { // level 2 start
favourite : { // level 3 start
sport : "football",
book : "Nothing"
},
RecentlyPlayed : {
game : "cricket",
videogame : "GTA5"
} // level 3 ends
} // level 2 ends
} // level 1 ends
} // level 0 ends
console.dir(object, {depth: null, showHidden: true, colors: true});
输出
正如我们在输出中看到的,我们得到了一种颜色用于对象的字符串属性,另一种颜色用于对象的整数属性。
firstname: 'James',
lastname: 'Anderson',
Initial: <ref *1> [Function: Initial] {
[length]: 0,
[name]: 'Initial',
[arguments]: null,
[caller]: null,
[prototype]: { [constructor]: [Circular *1] }
},
attrributes: {
age: 34,
school: 'High school',
hobbies: {
favourite: { sport: 'football', book: 'Nothing' },
RecentlyPlayed: { game: 'cricket', videogame: 'GTA5' }
}
}
}