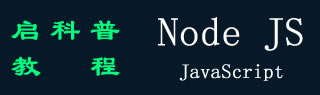
- Node.js教程
- Node.js - 教程
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 首次申请
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 活动
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 流程
- Node.js - 扩展应用程序
- Node.js - 包装
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲器
- Node.js - Streams
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL创建数据库
- Node.js - MySQL创建表
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where 子句
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - utility 模块
- Node.js - Web 模块
Node.js - Buffer.lastIndexOf() 方法
NodeJS Buffer.lastIndexOf() 方法负责在缓冲区内搜索值。它返回值存在的位置,如果值不存在,则返回 -1。如果缓冲区中多次出现该值,则将返回最后一个位置。
语法
以下是NodeJS lastIndexOf()方法的语法 -
buffer.lastIndexOf(value, byteoffset, encoding);
参数
buffer.lastIndexOf() 方法接受三个参数。第一个参数是必需的,其余参数是可选的。
- value − 这是一个强制性参数。它是要在缓冲区内搜索的值。该值可以是字符串、数字或缓冲区。
- byteOffset - 这是可选参数。它讲述了从哪里开始搜索的偏移量。如果给出负偏移值,它将从缓冲区的末端进行搜索。默认值为 buffer.length-1。
- encoding − 如果要搜索的值是字符串,则可以使用此参数给出编码。它是一个可选参数。默认情况下,使用的编码是 utf8。
返回值
Buffer.lastIndexOf() 方法将返回它遇到的搜索值的最后一个位置。如果它不存在,它将给出 -1。
例在此示例中,将尝试在创建的缓冲区内搜索值并测试结果。
const buffer = Buffer.from('Welcome to Qikepu');
if (buffer.lastIndexOf('Welcome') != -1) {
console.log("The string welcome is present in the buffer");
} else {
console.log("The string welcome is not present");
}
输出
我们正在使用字符串“Welcome to Qikepu”创建的缓冲区中搜索字符串 Welcome。由于给定的字符串存在,我们得到下面的输出。我们检查该值是否不等于 -1。因为当我们与缓冲区中给出的值没有任何匹配时,将返回 -1。
例
让我们尝试在下面的示例中给出 byteOffset 参数 -
const buffer = Buffer.from('Welcome to Qikepu');
if (buffer.lastIndexOf('Point', -1) != -1) {
console.log("The string point is present in the buffer");
} else {
console.log("The string point is not present");
}
输出
我们使用 byteOffset 作为 -1。它将从末尾搜索字符串“Welcome to Qikepu”。由于搜索字符串:Point 可用,您将获得打印为“字符串点存在于缓冲区中”的输出。
例
在此示例中,将使用缓冲区作为值来检查它在给定缓冲区内是否可用。
const buffer = Buffer.from('Welcome to Qikepu');
const result = buffer.lastIndexOf(Buffer.from('Qikepu'));
if (result != -1) {
console.log("The string Qikepu is present in the buffer");
} else {
console.log("The string Qikepu is not present");
}
输出
在 Buffer.lastIndexOf() 方法中,我们使用了一个具有字符串值的缓冲区:Qikepu。我们的主要缓冲区有一个字符串:Welcome to Qikepu。因此,由于字符串存在,因此返回的值是字符串的起始位置。
例
让我们尝试一个错误的情况,即在字符串不存在时检查 Buffer.lastIndexOf() 的响应。
const buffer = Buffer.from('Welcome to Qikepu');
if (buffer.lastIndexOf('Testing') != -1) {
console.log("The string Testing is present in the buffer");
} else {
console.log("The string Testing is not present");
}
输出
我们正在搜索字符串:使用字符串在缓冲区内进行测试:欢迎使用 Qikepu 。由于它不存在,因此它将返回值 -1。因此,您将得到以下输出: The string Testing is not present。
例
让我们测试一下 Buffer.lastIndexOf() 内部的编码参数。
const buffer = Buffer.from('Hello World');
if (buffer.lastIndexOf('Hello','hex') != -1) {
console.log("The string Hello is present in the buffer");
} else {
console.log("The string Hello is not present");
}
输出
我们使用了“十六进制”编码。当检查编码并且字符串存在时,它将返回字符串的起始位置。
例
在此示例中将使用整数值,即 utf-8 编码值在缓冲区中搜索。
const buffer = Buffer.from('Hello World');
if (buffer.lastIndexOf(72) != -1) {
console.log("The character H is present in the buffer");
} else {
console.log("The string H is not present");
}
输出
这里 72 代表字符“H”,按照 utf-8 编码。当在缓冲区中使用整数值进行搜索时,它返回字符 H 的位置,因为它存在于缓冲区中。
例
让我们看一个示例,其中同一字符串多次出现。
在示例中,我们使用 indexOf 和 lastIndexOf() 检查位置。使用 lastIndexOf() 它给出最后出现的字符串的位置,使用 indexOf() 它给出最先出现的字符串的位置。
const buffer = Buffer.from('She sells sea shells on the sea shore');
console.log("The position of sea using lastIndexOf() is "+ buffer.lastIndexOf("sea"));
console.log("The position of sea using indexOf() is "+ buffer.indexOf("sea"));
输出
The position of sea using indexOf() is 10