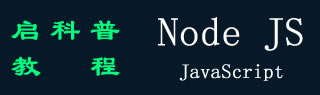
- Node.js教程
- Node.js - 教程
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 首次申请
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 活动
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 流程
- Node.js - 扩展应用程序
- Node.js - 包装
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲器
- Node.js - Streams
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL创建数据库
- Node.js - MySQL创建表
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where 子句
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - utility 模块
- Node.js - Web 模块
Node.js - doesNotMatch() 函数
Node.js assert.doesNotMatch() 函数将期望作为参数传递的 string 与将在第二个参数中传递给函数的 regexp 不匹配。
这是 Node.js 的 assert 模块的内置函数。
语法
以下是 assert.doesNotMatch() 函数的语法Node.js -
assert.doesNotMatch(string, regexp[, message]);
参数
此函数接受三个参数。下面将对此进行描述。
- string − (必需) 此参数仅在输入是字符串时才接受,否则它将向输出抛出 AssertionError。
- regexp − (必需) 在此参数中,我们需要传递一个不应与 string 匹配的 regexp 。如果两个输入( string 和 regexp )相同,它将向输出抛出 AssertionError。
- message − (可选)字符串或错误类型可以作为输入传递给此参数。
返回值
当两个输入( string 和 regexp )相同时,此方法将向输出抛出 AssertionError。
例在下面的示例中,我们将 string 和 regexp 值与 message 一起传递给Node.js assert.doesNotMatch() 函数。
const assert = require('assert');
var str = 'Qikepu';
var reg = /E-learning platform/;
assert.doesNotMatch(str, reg, 'Both are not matching');
输出
当我们编译并运行代码时,该函数不会向输出抛出任何 AssertionError,因为字符串和 regexp 都不相同。
例
在下面的示例中,我们将 string 和 regexp 值与 message 一起传递给Node.js assert.doesNotMatch() 函数。但是我们传递给 string 的值是一个整数。
const assert = require('assert');
var str = 96864;
var reg = /E-learning platform/;
assert.doesNotMatch(str, reg, 'Integer is passes instead of string');
输出
当我们编译并运行代码时,该函数会将 AssertionError 和 message 抛出到输出中,因为 String 输入值只能是字符串。
assert.doesNotMatch(str, reg, 'Integer is passes instead of string');
^
TypeError: assert.doesNotMatch is not a function
at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:6:8)
at Module._compile (internal/modules/cjs/loader.js:702:30)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10)
at Module.load (internal/modules/cjs/loader.js:612:32)
at tryModuleLoad (internal/modules/cjs/loader.js:551:12)
at Function.Module._load (internal/modules/cjs/loader.js:543:3)
at Function.Module.runMain (internal/modules/cjs/loader.js:744:10)
at startup (internal/bootstrap/node.js:238:19)
at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
例
在下面的示例中,我们将 String 和 regexp 传递给Node.js assert.doesNotMatch() 函数进行比较。但是我们还没有将任何文本传递给 message 参数。
const assert = require('assert');
var str = 436;
var reg = /Number/;
assert.doesNotMatch(str, reg);
输出
当我们编译并运行代码时,该函数将分配一个默认错误消息。
assert.doesNotMatch(str, reg);
^
TypeError: assert.doesNotMatch is not a function
at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:6:8)
at Module._compile (internal/modules/cjs/loader.js:702:30)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10)
at Module.load (internal/modules/cjs/loader.js:612:32)
at tryModuleLoad (internal/modules/cjs/loader.js:551:12)at Function.Module._load (internal/modules/cjs/loader.js:543:3)
at Function.Module.runMain (internal/modules/cjs/loader.js:744:10)
at startup (internal/bootstrap/node.js:238:19)
at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
例
在下面的示例中,我们将 string 和 regexp 值与消息一起传递给Node.js assert.doesNotMatch() 函数。
const assert = require('assert');
var str = 'Bahubali';
var reg = /Bahubali/;
assert.doesNotMatch(str, reg, 'Both the values are exactly same');
输出
当我们编译并运行代码时,该函数将向输出抛出 AssertionError,因为 string 和 regexp 都是相同的。
assert.doesNotMatch(str, reg, 'Both the values are exactly same');
^
TypeError: assert.doesNotMatch is not a function
at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:6:8)
at Module._compile (internal/modules/cjs/loader.js:702:30)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10)
at Module.load (internal/modules/cjs/loader.js:612:32)
at tryModuleLoad (internal/modules/cjs/loader.js:551:12)
at Function.Module._load (internal/modules/cjs/loader.js:543:3)
at Function.Module.runMain (internal/modules/cjs/loader.js:744:10)
at startup (internal/bootstrap/node.js:238:19)
at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
例
在下面的示例中,我们将 string 和 regexp 值与 message 一起传递给Node.js assert.doesNotMatch() 函数。
const assert = require('assert');
var str = 'Good to see you back officer';
var reg = /see/;
assert.doesNotMatch(str, reg, 'Both the values are partially same');
输出
当我们编译并运行代码时,该函数将向输出抛出 AssertionError,因为 string 和 regexp 部分相同。
assert.doesNotMatch(str, reg, 'Both the values are partially same');
^
TypeError: assert.doesNotMatch is not a function
at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:6:8)
at Module._compile (internal/modules/cjs/loader.js:702:30)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10)
at Module.load (internal/modules/cjs/loader.js:612:32)
at tryModuleLoad (internal/modules/cjs/loader.js:551:12)
at Function.Module._load (internal/modules/cjs/loader.js:543:3)
at Function.Module.runMain (internal/modules/cjs/loader.js:744:10)
at startup (internal/bootstrap/node.js:238:19)
at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)