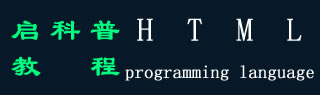
- HTML 教程
- HTML 教程
- HTML - 简介
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块和内联元素
- HTML - 样式表
- HTML - 文本格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图像
- HTML - 图像映射
- HTML - iframe
- HTML - 短语标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表格标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 电子邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB 和 RGBA 颜色
- HTML - 十六进制颜色
- HTML - HSL 和 HSLA 颜色
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 标头
- HTML - 头部
- HTML - 网站图标
- HTML - JavaScript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - CSS布局
- HTML - 响应式网页设计
- HTML - 特殊符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - 画布
- HTML API 接口
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSockets
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 数学标记语言
- HTML - 微观数据
- HTML - 索引数据库
- HTML - Web 消息传递
- HTML - CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - Web 幻灯片台
- HTML 工具
- HTML - 二维码
- HTML - Modernizr
- HTML - 验证器
- HTML 备忘单
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 代码参考
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- HTML - ISO 语言代码
- HTML - 字符编码
- HTML - 已弃用的标签和属性
HTML - CORS
跨域资源共享 (CORS) 是一种允许在 Web 浏览器中从另一个域加载受限资源的机制
例如,假设我们在 HTML5 演示部分单击 HTML5 视频播放器。首先,它会询问相机权限,如果用户允许权限,那么只有它会打开相机,否则不会。
提出 CORS 请求
Chrome、Firefox、Opera 和 Safari 等现代浏览器都使用 XMLHttprequest2 对象,而 Internet Explorer 使用类似的 XDomainRequest 对象。
function createCORSRequest(method, url) {
var xhr = new XMLHttpRequest();
if ("withCredentials" in xhr) {
// Check if the XMLHttpRequest object has a "withCredentials" property.
// "withCredentials" only exists on XMLHTTPRequest2 objects.
xhr.open(method, url, true);
} else if (typeof XDomainRequest != "undefined") {
// Otherwise, check if XDomainRequest.
// XDomainRequest only exists in IE, and is IE's way of making CORS requests.
xhr = new XDomainRequest();
xhr.open(method, url);
}
else {
// Otherwise, CORS is not supported by the browser.
xhr = null;
}
return xhr;
}
var xhr = createCORSRequest('GET', url);
if (!xhr) {
throw new Error('CORS not supported');
}
CORS 中的事件句柄
事件处理程序 | 描述 |
---|---|
onloadstart |
启动请求 |
onprogress |
加载数据并发送数据 |
onabort |
中止请求 |
onerror |
请求失败 |
onload |
请求加载成功 |
ontimeout |
在请求完成之前已超时 |
onloadend |
当请求完成时,成功或失败 |
onload 或 onerror 事件的示例
xhr.onload = function() {
var responseText = xhr.responseText;
// process the response.
console.log(responseText);
};
xhr.onerror = function() {
console.log('There was an error!');
};
带有处理程序的 CORS 示例
下面的示例将显示 makeCorsRequest() 和 onload 处理程序的示例
// Create the XHR object.
function createCORSRequest(method, url) {
var xhr = new XMLHttpRequest();
if ("withCredentials" in xhr) {
// XHR for Chrome/Firefox/Opera/Safari.
xhr.open(method, url, true);
} else if (typeof XDomainRequest != "undefined") {
// XDomainRequest for IE.
xhr = new XDomainRequest();
xhr.open(method, url);
} else {
// CORS not supported.
xhr = null;
}
return xhr;
}
// Helper method to parse the title tag from the response.
function getTitle(text) {
return text.match('<title>(.*)?</title>')[1];
}
// Make the actual CORS request.
function makeCorsRequest() {
// All HTML5 Rocks properties support CORS.
var url = 'http://www.qikepu.com';
var xhr = createCORSRequest('GET', url);
if (!xhr) {
alert('CORS not supported');
return;
}
// Response handlers.
xhr.onload = function() {
var text = xhr.responseText;
var title = getTitle(text);
alert('Response from CORS request to ' + url + ': ' + title);
};
xhr.onerror = function() {
alert('Woops, there was an error making the request.');
};
xhr.send();
}