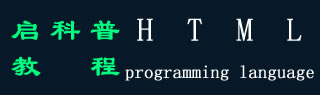
- HTML 教程
- HTML 教程
- HTML - 简介
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块和内联元素
- HTML - 样式表
- HTML - 文本格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图像
- HTML - 图像映射
- HTML - iframe
- HTML - 短语标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表格标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 电子邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB 和 RGBA 颜色
- HTML - 十六进制颜色
- HTML - HSL 和 HSLA 颜色
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 标头
- HTML - 头部
- HTML - 网站图标
- HTML - JavaScript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - CSS布局
- HTML - 响应式网页设计
- HTML - 特殊符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - 画布
- HTML API 接口
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSockets
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 数学标记语言
- HTML - 微观数据
- HTML - 索引数据库
- HTML - Web 消息传递
- HTML - CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - Web 幻灯片台
- HTML 工具
- HTML - 二维码
- HTML - Modernizr
- HTML - 验证器
- HTML 备忘单
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 代码参考
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- HTML - ISO 语言代码
- HTML - 字符编码
- HTML - 已弃用的标签和属性
HTML - 画布
HTML 元素 <canvas> 为您提供了一种使用 JavaScript 绘制图形的简单而强大的方法。它可以用来绘制图表、制作照片构图或制作简单(和不那么简单)的动画。
下面是一个简单的 <canvas> 元素,它只有两个特定的属性 width 和 height 以及所有核心 HTML 属性,如 id、name 和 class 等。
<canvas id="mycanvas" width="100" height="100"></canvas>
您可以使用 getElementById() 方法在 DOM 中轻松找到 <canvas> 元素,如下所示:
var canvas = document.getElementById("mycanvas");
例子
让我们看一个示例,它显示了如何在 HTML 文档中使用 <canvas> 元素。
<!DOCTYPE html>
<html>
<head>
<style>
#mycanvas{border:1px solid red;}
</style>
</head>
<body>
<canvas id="mycanvas" width="100" height="100"></canvas>
</body>
</html>
渲染上下文
<canvas> 最初是空白的,要显示某些内容,脚本首先需要访问渲染上下文。canvas 元素具有一个名为 getContext 的 DOM 方法,该方法用于获取渲染上下文及其绘图函数。此函数采用一个参数,即上下文类型 2d。
实例
以下是获取所需上下文的代码,以及检查浏览器是否支持 <canvas> 元素:
var canvas = document.getElementById("mycanvas");
if (canvas.getContext){
var ctx = canvas.getContext('2d');
// drawing code here
} else {
// canvas-unsupported code here
}
浏览器支持
最新版本的 Firefox、Safari、Chrome 和 Opera 都支持 HTML Canvas,但 IE8 本身不支持 Canvas。
可以使用 ExplorerCanvas 通过 Internet Explorer 获得画布支持。你只需要包含这个JavaScript,如下所示 -
<!--[if IE]>
<script src="excanvas.js"></script>
<![endif]-->
HTML Canvas - 绘制矩形
有三种方法可以在画布上绘制矩形:
方法 | 描述 |
---|---|
fillRect(x,y,width,height) |
此方法绘制一个填充的矩形。 |
strokeRect(x,y,width,height) |
此方法绘制矩形轮廓。 |
clearRect(x,y,width,height) |
此方法清除指定区域并使其完全透明 |
此处,x 和 y 指定矩形左上角在画布上的位置(相对于原点),宽度和高度是矩形的宽度和高度。
例子
下面是一个简单的例子,它利用上面提到的方法来绘制一个漂亮的矩形。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// Get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
// Draw shapes
ctx.fillRect(25,25,100,100);
ctx.clearRect(45,45,60,60);
ctx.strokeRect(50,50,50,50);
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
HTML Canvas - 绘图路径
我们需要以下方法在画布上绘制路径:
方法 | 描述 |
---|---|
beginPath() |
此方法重置当前路径。 |
moveTo(x, y) |
此方法使用给定点创建新的子路径。 |
closePath() |
此方法将当前子路径标记为闭合,并启动新子路径,其点与新闭合子路径的起点和终点相同。 |
fill () |
此方法使用当前填充样式填充子路径。 |
stroke() |
此方法使用当前描边样式描边子路径。 |
arc(x, y, radius, startAngle, endAngle, anticlockwise) |
向子路径添加点,使得由参数描述的圆的周长描述的圆弧(从给定的起始角度开始,到给定的结束角度结束,沿给定方向前进)被添加到路径中,通过直线连接到前一个点。 |
例子
下面是一个简单的例子,它利用上面提到的方法来绘制一个形状。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
// Draw shapes
ctx.beginPath();
ctx.arc(75,75,50,0,Math.PI*2,true); // Outer circle
ctx.moveTo(110,75);
ctx.arc(75,75,35,0,Math.PI,false); // Mouth
ctx.moveTo(65,65);
ctx.arc(60,65,5,0,Math.PI*2,true); // Left eye
ctx.moveTo(95,65);
ctx.arc(90,65,5,0,Math.PI*2,true); // Right eye
ctx.stroke();
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
HTML Canvas - 画线
Line 方法
我们需要以下方法在画布上画线:
方法 | 描述 |
---|---|
beginPath() |
此方法重置当前路径。 |
moveTo(x, y) |
此方法使用给定点创建新的子路径。 |
closePath() |
此方法将当前子路径标记为闭合,并启动新子路径,其点与新闭合子路径的起点和终点相同。 |
fill() |
此方法使用当前填充样式填充子路径。 |
stroke() |
此方法使用当前描边样式描边子路径。 |
lineTo(x, y) |
此方法将给定点添加到当前子路径中,通过直线连接到前一个子路径。 |
例子
下面是一个简单的例子,它利用上述方法绘制一个三角形。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
// Filled triangle
ctx.beginPath();
ctx.moveTo(25,25);
ctx.lineTo(105,25);
ctx.lineTo(25,105);
ctx.fill();
// Stroked triangle
ctx.beginPath();
ctx.moveTo(125,125);
ctx.lineTo(125,45);
ctx.lineTo(45,125);
ctx.closePath();
ctx.stroke();
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
HTML Canvas - 绘制贝塞尔曲线
我们需要以下方法在画布上绘制贝塞尔曲线:
方法 | 描述 |
---|---|
beginPath() |
此方法重置当前路径。 |
moveTo(x, y) |
此方法使用给定点创建新的子路径。 |
closePath() |
此方法将当前子路径标记为闭合,并启动新子路径,其点与新闭合子路径的起点和终点相同。 |
fill() |
此方法使用当前填充样式填充子路径。 |
stroke() |
此方法使用当前描边样式描边子路径。 |
bezierCurveTo(cp1x, cp1y, cp2x, cp2y, x, y) |
此方法将给定点添加到当前路径中,通过具有给定控制点的立方贝塞尔曲线连接到前一个路径。 |
bezierCurveTo() 方法中的 x 和 y 参数是终点的坐标。CP1x和CP1Y为第一控制点的坐标,CP2X和CP2Y为第二控制点的坐标。
例子
下面是一个简单的例子,它利用上面提到的方法来绘制贝塞尔曲线。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
ctx.beginPath();
ctx.moveTo(75,40);
ctx.bezierCurveTo(75,37,70,25,50,25);
ctx.bezierCurveTo(20,25,20,62.5,20,62.5);
ctx.bezierCurveTo(20,80,40,102,75,120);
ctx.bezierCurveTo(110,102,130,80,130,62.5);
ctx.bezierCurveTo(130,62.5,130,25,100,25);
ctx.bezierCurveTo(85,25,75,37,75,40);
ctx.fill();
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
HTML Canvas - 绘制二次曲线
我们需要以下方法在画布上绘制二次曲线:
方法 | 描述 |
---|---|
beginPath() |
此方法重置当前路径。 |
moveTo(x, y) |
此方法使用给定点创建新的子路径。 |
closePath() |
此方法将当前子路径标记为闭合,并启动新子路径,其点与新闭合子路径的起点和终点相同。 |
fill () |
此方法使用当前填充样式填充子路径。 |
stroke () |
此方法使用当前描边样式描边子路径。 |
quadraticCurveTo(cpx, cpy, x, y) |
此方法将给定点添加到当前路径中,通过具有给定控制点的二次贝塞尔曲线连接到前一个路径。 |
quadraticCurveTo() 方法中的 x 和 y 参数是终点的坐标。cpx 和 cpy 是控制点的坐标。
例子
下面是一个简单的例子,它利用上述方法来绘制二次曲线。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
// Draw shapes
ctx.beginPath();
ctx.moveTo(75,25);
ctx.quadraticCurveTo(25,25,25,62.5);
ctx.quadraticCurveTo(25,100,50,100);
ctx.quadraticCurveTo(50,120,30,125);
ctx.quadraticCurveTo(60,120,65,100);
ctx.quadraticCurveTo(125,100,125,62.5);
ctx.quadraticCurveTo(125,25,75,25);
ctx.stroke();
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
HTML Canvas - 使用图像
本教程将展示如何将外部图像导入画布,然后如何使用以下方法在该图像上绘图:
方法 | 描述 |
---|---|
beginPath() |
此方法重置当前路径。 |
moveTo(x, y) |
此方法使用给定点创建新的子路径。 |
closePath() |
此方法将当前子路径标记为闭合,并启动新子路径,其点与新闭合子路径的起点和终点相同。 |
fill () |
此方法使用当前填充样式填充子路径。 |
stroke () |
此方法使用当前描边样式描边子路径。 |
drawImage(image, dx, dy) |
此方法将给定的图像绘制到画布上。这里的 image 是对图像或画布对象的引用。x 和 y 构成了目标画布上的坐标,我们的图像应该被放置在那里。 |
例子
下面是一个简单的示例,它使用上述方法来导入图像。
<!DOCTYPE HTML>
<html>
<head>
<script type = "text/javascript">
function drawShape() {
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext) {
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
// Draw shapes
var img = new Image();
img.src = '/html/images/backdrop.jpg';
img.onload = function() {
ctx.drawImage(img,0,0);
ctx.beginPath();
ctx.moveTo(30,96);
ctx.lineTo(70,66);
ctx.lineTo(103,76);
ctx.lineTo(170,15);
ctx.stroke();
}
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body onload = "drawShape();">
<canvas id = "mycanvas"></canvas>
</body>
</html>
HTML Canvas - 创建渐变
HTML 画布允许我们使用以下方法使用线性和径向渐变填充和描边形状:
方法 | 描述 |
---|---|
addColorStop(offset, color) |
此方法将具有给定颜色的色标添加到给定偏移处的渐变中。这里 0.0 是梯度一端的偏移量,1.0 是另一端的偏移量。 |
createLinearGradient(x0, y0, x1, y1) |
此方法返回一个 CanvasGradient 对象,该对象表示沿参数表示的坐标给出的线绘制的线性渐变。这四个参数表示梯度的起点 (x1,y1) 和终点 (x2,y2)。 |
createRadialGradient(x0, y0, r0, x1, y1, r1) |
此方法返回一个 CanvasGradient 对象,该对象表示径向渐变,该径向渐变沿参数表示的圆给出的圆锥体绘制。前三个参数定义一个坐标为 (x1,y1) 和半径 r1 的圆,第二个参数定义一个坐标为 (x2,y2) 和半径 r2 的圆。 |
示例 - 线性梯度
下面是一个简单的示例,它利用上述方法来创建线性梯度。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width:100px;
height:100px;
margin:0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
// Create Linear Gradients
var lingrad = ctx.createLinearGradient(0,0,0,150);
lingrad.addColorStop(0, '#00ABEB');
lingrad.addColorStop(0.5, '#fff');
lingrad.addColorStop(0.5, '#66CC00');
lingrad.addColorStop(1, '#fff');
var lingrad2 = ctx.createLinearGradient(0,50,0,95);
lingrad2.addColorStop(0.5, '#000');
lingrad2.addColorStop(1, 'rgba(0,0,0,0)');
// assign gradients to fill and stroke styles
ctx.fillStyle = lingrad;
ctx.strokeStyle = lingrad2;
// draw shapes
ctx.fillRect(10,10,130,130);
ctx.strokeRect(50,50,50,50);
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
示例 - 径向梯度
下面是一个简单的示例,它利用上述方法来创建径向渐变。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height: 100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape() {
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext) {
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
// Create gradients
var radgrad = ctx.createRadialGradient(45, 45, 10, 52, 50, 30);
radgrad.addColorStop(0, '#A7D30C');
radgrad.addColorStop(0.9, '#019F62');
radgrad.addColorStop(1, 'rgba(1,159,98,0)');
var radgrad2 = ctx.createRadialGradient(105, 105, 20, 112, 120, 50);
radgrad2.addColorStop(0, '#FF5F98');
radgrad2.addColorStop(0.75, '#FF0188');
radgrad2.addColorStop(1, 'rgba(255,1,136,0)');
var radgrad3 = ctx.createRadialGradient(95, 15, 15, 102, 20, 40);
radgrad3.addColorStop(0, '#00C9FF');
radgrad3.addColorStop(0.8, '#00B5E2');
radgrad3.addColorStop(1, 'rgba(0,201,255,0)');
var radgrad4 = ctx.createRadialGradient(0, 150, 50, 0, 140, 90);
radgrad4.addColorStop(0, '#F4F201');
radgrad4.addColorStop(0.8, '#E4C700');
radgrad4.addColorStop(1, 'rgba(228,199,0,0)');
// draw shapes
ctx.fillStyle = radgrad4;
ctx.fillRect(0, 0, 150, 150);
ctx.fillStyle = radgrad3;
ctx.fillRect(0, 0, 150, 150);
ctx.fillStyle = radgrad2;
ctx.fillRect(0, 0, 150, 150);
ctx.fillStyle = radgrad;
ctx.fillRect(0, 0, 150, 150);
}
else {
alert('您需要Safari或Firefox 1.5+才能观看此演示。');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
HTML Canvas - 样式和颜色
HTML canvas 提供以下两个重要属性来将颜色应用于形状:
方法 | 描述 |
---|---|
fillStyle |
此属性表示要在形状中使用的颜色或样式。 |
strokeStyle |
此属性表示用于形状周围线条的颜色或样式 |
默认情况下,描边和填充颜色设置为黑色,即 CSS 颜色值 #000000。
示例 - fillStyle
下面是一个简单的示例,它利用上面提到的 fillStyle 属性来创建一个漂亮的模式。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
// Create a pattern
for (var i=0;i<7;i++){
for (var j=0;j<7;j++){
ctx.fillStyle='rgb(' + Math.floor(255-20.5*i)+ ','+ Math.floor(255 - 42.5*j) + ',255)';
ctx.fillRect( j*25, i* 25, 55, 55 );
}
}
}
else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
示例 - strokeStyle
下面是一个简单的示例,它利用上面提到的 fillStyle 属性来创建另一个漂亮的模式。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
// Create a pattern
for (var i=0;i<10;i++){
for (var j=0;j<10;j++){
ctx.strokeStyle='rgb(255,'+ Math.floor(50-2.5*i)+','+ Math.floor(155 - 22.5 * j ) + ')';
ctx.beginPath();
ctx.arc(1.5+j*25, 1.5 + i*25,10,10,Math.PI*5.5, true);
ctx.stroke();
}
}
}
else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
HTML Canvas - 文本和字体
HTML canvas 提供了使用下面列出的不同字体和文本属性创建文本的功能:
S.No。 | 属性和描述 |
---|---|
1 |
font [ = value ] 此属性返回当前字体设置,可以设置以更改字体。 |
2 |
textAlign [ = value ] 此属性返回当前文本对齐设置,可以设置此属性以更改对齐方式。可能的值为 start、end、left、right 和 center。 |
3 |
textBaseline [ = value ] 此属性返回当前基线对齐设置,可以设置此属性以更改基线对齐方式。可能的值为 top、hanging、middle、alphabeticic、ideographic 和 bottom |
4 |
fillText(text, x, y [, maxWidth ] ) 此属性在给定坐标 x 和 y 指示的给定位置填充给定文本。 |
5 |
strokeText(text, x, y [, maxWidth ] ) 此属性在给定坐标 x 和 y 指示的给定位置描边给定文本。 |
例子
下面是一个简单的例子,它利用上面提到的属性来绘制文本:
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
ctx.fillStyle = '#00F';
ctx.font = 'Italic 30px Sans-Serif';
ctx.textBaseline = 'Top';
ctx.fillText ('Hello world!', 40, 100);
ctx.font = 'Bold 30px Sans-Serif';
ctx.strokeText('Hello world!', 40, 50);
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
HTML Canvas - 图案和阴影
创建图案
在画布上创建图案需要以下方法:
S.No。 | 方法和描述 |
---|---|
1 |
createPattern(image, repetition) 此方法将使用 image 创建模式。第二个参数可以是具有以下值之一的字符串:repeat、repeat-x、repeat-y 和 no-repeat。如果指定了空字符串或 null,则重复 will。假设 |
例子
下面是一个简单的例子,它利用上面提到的方法来创建一个漂亮的模式。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width:100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
// create new image object to use as pattern
var img = new Image();
img.src = '/html/images/pattern.jpg';
img.onload = function(){
// create pattern
var ptrn = ctx.createPattern(img,'repeat');
ctx.fillStyle = ptrn;
ctx.fillRect(0,0,150,150);
}
}
else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
假设我们有以下模式:

创建阴影
HTML 画布提供了在绘图周围创建漂亮阴影的功能。所有绘图操作都受四个全局阴影属性的影响。
S.No。 | 属性和描述 |
---|---|
1 |
shadowColor [ = value ] 此属性返回当前阴影颜色,可以设置此属性以更改阴影颜色。 |
2 |
shadowOffsetX [ = value ] 此属性返回当前阴影偏移量 X,并且可以设置此属性以更改阴影偏移量 X。 |
3 |
shadowOffsetY [ = value ] 此属性返回当前阴影偏移量 Y,并且可以设置、更改阴影偏移量 Y。 |
4 |
shadowBlur [ = value ] 此属性返回应用于阴影的当前模糊级别,可以设置此属性以更改模糊级别。 |
例子
下面是一个简单的示例,它利用上述属性来绘制阴影。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
ctx.shadowOffsetX = 2;
ctx.shadowOffsetY = 2;
ctx.shadowBlur = 2;
ctx.shadowColor = "rgba(0, 0, 0, 0.5)";
ctx.font = "20px Times New Roman";
ctx.fillStyle = "Black";
ctx.fillText("This is shadow test", 5, 30);
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
HTML Canvas - 保存和恢复状态
HTML canvas 提供了两种重要的方法来保存和恢复画布状态。画布绘图状态基本上是已应用的所有样式和转换的快照,由以下内容组成 -
- 转换,如平移、旋转和缩放等。
- 当前剪切区域。
- 以下属性的当前值 - strokeStyle、fillStyle、globalAlpha、lineWidth、lineCap、lineJoin、miterLimit、shadowOffsetX、shadowOffsetY、shadowBlur、shadowColor、globalCompositeOperation、font、textAlign、textBaseline。
每次调用 save 方法时,画布状态都会存储在堆栈上,每次调用 restore 方法时,都会从堆栈中返回上次保存的状态。
S.No。 | 方法和描述 |
---|---|
1 |
save() 此方法将当前状态推送到堆栈上。 |
2 |
restore() 此方法在堆栈上弹出顶部状态,将上下文还原到该状态。 |
例子
下面是一个简单的示例,它利用上述方法来显示如何调用还原,以恢复原始状态,并再次将最后一个矩形绘制为黑色。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
// draw a rectangle with default settings
ctx.fillRect(0,0,150,150);
// Save the default state
ctx.save();
// Make changes to the settings
ctx.fillStyle = '#66FFFF'
ctx.fillRect( 15,15,120,120);
// Save the current state
ctx.save();
// Make the new changes to the settings
ctx.fillStyle = '#993333'
ctx.globalAlpha = 0.5;
ctx.fillRect(30,30,90,90);
// Restore previous state
ctx.restore();
// Draw a rectangle with restored settings
ctx.fillRect(45,45,60,60);
// Restore original state
ctx.restore();
// Draw a rectangle with restored settings
ctx.fillRect(40,40,90,90);
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas"></canvas>
</body>
</html>
HTML Canvas - 翻译
HTML canvas 提供了 translate(x, y) 方法,用于将画布及其原点移动到网格中的不同点。
这里的参数 x 是画布向左或向右移动的量,y 是它向上或向下移动的量
例子
下面是一个简单的例子,它利用上述方法绘制各种Spirographs——
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width:100px;
height:100px;
margin:0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
ctx.fillRect(0,0,300,300);
for (i=0;i<3;i++) {
for (j=0;j<3;j++) {
ctx.save();
ctx.strokeStyle = "#FF0066";
ctx.translate(50+j*100,50+i*100);
drawSpirograph(ctx,10*(j+3)/(j+2),-2*(i+3)/(i+1),10);
ctx.restore();
}
}
}
else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
function drawSpirograph(ctx,R,r,O){
var x1 = R-O;
var y1 = 0;
var i = 1;
ctx.beginPath();
ctx.moveTo(x1,y1);
do {
if (i>20000) break;
var x2 = (R+r)*Math.cos(i*Math.PI/72) - (r+O)*Math.cos(((R+r)/r)*(i*Math.PI/72))
var y2 = (R+r)*Math.sin(i*Math.PI/72) - (r+O)*Math.sin(((R+r)/r)*(i*Math.PI/72))
ctx.lineTo(x2,y2);
x1 = x2;
y1 = y2;
i++;
} while (x2 != R-O && y2 != 0 );
ctx.stroke();
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas" width="400" height="400"></canvas>
</body>
</html>
HTML Canvas - 旋转
HTML canvas 提供了 rotate(angle) 方法,用于围绕当前原点旋转画布。
此方法仅采用一个参数,即画布旋转的角度。这是以弧度为单位的顺时针旋转。
例子
下面是一个简单的例子,我们正在运行两个循环,其中第一个循环决定了环的数量,第二个循环决定了每个环中绘制的点数。
<!DOCTYPE html>
<html>
<head>
<style>
#test {
width: 100px;
height:100px;
margin: 0px auto;
}
</style>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
ctx.translate(100,100);
for (i=1; i<7; i++){
ctx.save();
ctx.fillStyle = 'rgb('+(51*i)+','+(200-51*i)+',0)';
for (j=0; j < i*6; j++){
ctx.rotate(Math.PI*2/(i*6));
ctx.beginPath();
ctx.arc(0,i*12.5,5,0,Math.PI*2,true);
ctx.fill();
}
ctx.restore();
}
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body id="test" onload="drawShape();">
<canvas id="mycanvas" width="400" height="400"></canvas>
</body>
</html>
HTML Canvas - 缩放
HTML canvas 提供了 scale(x, y) 方法,用于增加或减少 canvas 网格中的单位。这可用于绘制缩小或放大的形状和位图。
此方法采用两个参数,其中 x 是水平方向的比例因子,y 是垂直方向的比例因子。这两个参数都必须是正数。
小于 1.0 的值会减小单位大小,大于 1.0 的值会增加单位大小。将比例因子精确设置为 1.0 不会影响单位大小。
例子
下面是一个简单的例子,它使用肺活量计功能绘制了九个具有不同比例因子的形状。
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript">
function drawShape(){
// Get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// Use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
ctx.strokeStyle = "#fc0";
ctx.lineWidth = 1.5;
ctx.fillRect(0,0,300,300);
// Uniform scaling
ctx.save()
ctx.translate(50,50);
drawSpirograph(ctx,22,6,5);
ctx.translate(100,0);
ctx.scale(0.75,0.75);
drawSpirograph(ctx,22,6,5);
ctx.translate(133.333,0);
ctx.scale(0.75,0.75);
drawSpirograph(ctx,22,6,5);
ctx.restore();
// Non uniform scaling (y direction)
ctx.strokeStyle = "#0cf";
ctx.save()
ctx.translate(50,150);
ctx.scale(1,0.75);
drawSpirograph(ctx,22,6,5);
ctx.translate(100,0);
ctx.scale(1,0.75);
drawSpirograph(ctx,22,6,5);
ctx.translate(100,0);
ctx.scale(1,0.75);
drawSpirograph(ctx,22,6,5);
ctx.restore();
// Non uniform scaling (x direction)
ctx.strokeStyle = "#cf0";
ctx.save()
ctx.translate(50,250);
ctx.scale(0.75,1);
drawSpirograph(ctx,22,6,5);
ctx.translate(133.333,0);
ctx.scale(0.75,1);
drawSpirograph(ctx,22,6,5);
ctx.translate(177.777,0);
ctx.scale(0.75,1);
drawSpirograph(ctx,22,6,5);
ctx.restore();
} else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
function drawSpirograph(ctx,R,r,O){
var x1 = R-O;
var y1 = 0;
var i = 1;
ctx.beginPath();
ctx.moveTo(x1,y1);
do {
if (i>20000) break;
var x2 = (R+r)*Math.cos(i*Math.PI/72) - (r+O)*Math.cos(((R+r)/r)*(i*Math.PI/72))
var y2 = (R+r)*Math.sin(i*Math.PI/72) - (r+O)*Math.sin(((R+r)/r)*(i*Math.PI/72))
ctx.lineTo(x2,y2);
x1 = x2;
y1 = y2;
i++;
}
while (x2 != R-O && y2 != 0 );
ctx.stroke();
}
</script>
</head>
<body onload="drawShape();">
<canvas id="mycanvas" width="400" height="400"></canvas>
</body>
</html>
HTML Canvas - 转换
HTML canvas 提供了允许直接修改转换矩阵的方法。转换矩阵最初必须是标识转换。然后可以使用转换方法对其进行调整。
S.No。 | 方法和描述 |
---|---|
1 |
transform(m11, m12, m21, m22, dx, dy) 此方法更改转换矩阵以应用参数给出的矩阵。 |
2 |
setTransform(m11, m12, m21, m22, dx, dy) 此方法将变换矩阵更改为参数给出的矩阵。 |
变换 (m11, m12, m21, m22, dx, dy) 方法必须将当前变换矩阵乘以 −
m12 m22 dy
0 0 1
setTransform(m11, m12, m21, m22, dx, dy) 方法必须将当前变换重置为单位矩阵,然后使用相同的参数调用 transform(m11, m12, m21, m22, dx, dy) 方法。
例子下面是一个使用 transform() 和 setTransform() 方法的简单示例 −
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript">
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
var sin = Math.sin(Math.PI/6);
var cos = Math.cos(Math.PI/6);
ctx.translate(200, 200);
var c = 0;
for (var i=0; i <= 12; i++) {
c = Math.floor(255 / 12 * i);
ctx.fillStyle = "rgb(" + c + "," + c + "," + c + ")";
ctx.fillRect(0, 0, 100, 100);
ctx.transform(cos, sin, -sin, cos, 0, 0);
}
ctx.setTransform(-1, 0, 0, 1, 200, 200);
ctx.fillStyle = "rgba(100, 100, 255, 0.5)";
ctx.fillRect(50, 50, 100, 100);
}
else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body onload="drawShape();">
<canvas id="mycanvas" width="400" height="400"></canvas>
</body>
</html>
HTML Canvas - 组合
HTML canvas 提供影响所有绘图操作的合成属性 globalCompositeOperation。
我们可以在现有形状后面绘制新形状并遮罩某些区域,使用 globalCompositeOperation 属性从画布上清除部分,如下图所示。
可以为 globalCompositeOperation 设置以下值 :
S.No。 | 属性和描述 |
---|---|
1 |
source-over 这是默认设置,可在现有画布内容上绘制新形状。 |
2 |
source-in 仅当新形状和目标画布重叠时,才会绘制新形状。其他一切都是透明的。 |
3 |
source-out 新形状的绘制位置与现有画布内容不重叠。 |
4 |
source-atop 仅绘制新形状与现有画布内容重叠的位置。 |
5 |
lighter 如果两个形状重叠,则通过添加颜色值来确定颜色。 |
6 |
xor 形状在重叠的地方变得透明,并在其他任何地方绘制正常。 |
7 |
destination-over 新形状绘制在现有画布内容后面。 |
8 |
destination-in 现有画布内容将保留在新形状和现有画布内容重叠的地方。其他一切都是透明的。 |
9 |
destination-out 现有内容将保留在不与新形状重叠的位置。 |
10 |
destination-atop 现有画布仅保留在与新形状重叠的地方。新形状绘制在画布内容后面。 |
11 |
darker 如果两个形状重叠,则通过减去颜色值来确定颜色。 |
例子
下面是一个简单的示例,它利用 globalCompositeOperation 属性创建所有可能的组合:
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript">
var compositeTypes = [
'source-over','source-in','source-out','source-atop',
'destination-over','destination-in','destination-out',
'destination-atop','lighter','darker','copy','xor'
];
function drawShape(){
for (i=0;i<compositeTypes.length;i++){
var label = document.createTextNode(compositeTypes[i]);
document.getElementById('lab'+i).appendChild(label);
var ctx = document.getElementById('tut'+i).getContext('2d');
// draw rectangle
ctx.fillStyle = "#FF3366";
ctx.fillRect(15,15,70,70);
// set composite property
ctx.globalCompositeOperation = compositeTypes[i];
// draw circle
ctx.fillStyle = "#0066FF";
ctx.beginPath();
ctx.arc(75,75,35,0,Math.PI*2,true);
ctx.fill();
}
}
</script>
</head>
<body onload="drawShape();">
<table border="1" align="center">
<tr>
<td><canvas id="tut0" width="125" height="125"></canvas><br/>
<label id="lab0"></label>
</td>
<td><canvas id="tut1" width="125" height="125"></canvas><br/>
<label id="lab1"></label>
</td>
<td><canvas id="tut2" width="125" height="125"></canvas><br/>
<label id="lab2"></label>
</td>
</tr>
<tr>
<td><canvas id="tut3" width="125" height="125"></canvas><br/>
<label id="lab3"></label>
</td>
<td><canvas id="tut4" width="125" height="125"></canvas><br/>
<label id="lab4"></label>
</td>
<td><canvas id="tut5" width="125" height="125"></canvas><br/>
<label id="lab5"></label>
</td>
</tr>
<tr>
<td><canvas id="tut6" width="125" height="125"></canvas><br/>
<label id="lab6"></label>
</td>
<td><canvas id="tut7" width="125" height="125"></canvas><br/>
<label id="lab7"></label>
</td>
<td><canvas id="tut8" width="125" height="125"></canvas><br/>
<label id="lab8"></label>
</td>
</tr>
<tr>
<td><canvas id="tut9" width="125" height="125"></canvas><br/>
<label id="lab9"></label>
</td>
<td><canvas id="tut10" width="125" height="125"></canvas><br/>
<label id="lab10"></label>
</td>
<td><canvas id="tut11" width="125" height="125"></canvas><br/>
<label id="lab11"></label>
</td>
</tr>
</table>
</body>
</html>
HTML Canvas - 动画
HTML canvas 提供了绘制图像并完全擦除图像的必要方法。我们可以借助 Javascript 在 HTML 画布上模拟良好的动画。
以下是两种重要的 Javascript 方法,它们将用于在画布上为图像制作动画:
S.No。 | 方法和描述 |
---|---|
1 |
setInterval(callback, time); 此方法在给定的时间毫秒后重复执行提供的代码。 |
2 |
setTimeout(callback, time); 此方法仅在给定时间毫秒后执行一次提供的代码。 |
例子
下面是一个重复旋转小图像的简单示例:
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript">
var pattern= new Image();
function animate(){
pattern.src = '/html/images/pattern.jpg';
setInterval(drawShape, 100);
}
function drawShape(){
// get the canvas element using the DOM
var canvas = document.getElementById('mycanvas');
// Make sure we don't execute when canvas isn't supported
if (canvas.getContext){
// use getContext to use the canvas for drawing
var ctx = canvas.getContext('2d');
ctx.fillStyle = 'rgba(0,0,0,0.4)';
ctx.strokeStyle = 'rgba(0,153,255,0.4)';
ctx.save();
ctx.translate(150,150);
var time = new Date();
ctx.rotate( ((2*Math.PI)/6)*time.getSeconds() + ( (2*Math.PI)/6000)*time.getMilliseconds() );
ctx.translate(0,28.5);
ctx.drawImage(pattern,-3.5,-3.5);
ctx.restore();
}
else {
alert('You need Safari or Firefox 1.5+ to see this demo.');
}
}
</script>
</head>
<body onload="animate();">
<canvas id="mycanvas" width="400" height="400"></canvas>
</body>
</html>