提供发送电子邮件是典型的 PHP 驱动的 Web 应用程序通常需要的功能之一。您希望通过 PHP 应用程序本身而不是其他邮件服务向注册用户发送包含通知、更新和其他通信的电子邮件。您可以通过采用本章中描述的技术将此功能添加到您的 PHP 应用程序中。
PHP 有一个内置的 mail() 函数来发送电子邮件。但是,您需要正确配置 “php.ini” 设置才能执行此操作。首先,您必须知道您正在使用的 Web 托管平台的 SMTP 域。例如,如果您的网站托管在 GoDaddy 托管服务上,则 SMTP 域为“smtp.secureserver.net”,您应该在配置中使用该域。
如果您使用基于 Windows 的 GoDaddy 托管,则应确保在 php.ini 文件中启用了两个指令。第一个称为 SMTP,用于定义您的电子邮件服务器地址。第二个称为 sendmail_from,定义您自己的电子邮件地址。
Windows 的配置应如下所示 -
; For Win32 only.
SMTP = smtp.secureserver.net
; For win32 only
sendmail_from = webmaster@qikepu.com
Linux 用户只需让 PHP 知道他们的 sendmail 应用程序的位置。path 和任何所需的交换都应该指定给 sendmail_path 指令。
Linux 的配置应如下所示 -
; For Win32 only.
SMTP =
; For win32 only
sendmail_from =
; For Unix only
sendmail_path = /usr/sbin/sendmail -t -i
php 的 mail() 函数需要三个强制性参数,分别指定收件人的电子邮件地址、消息的主题和实际消息,此外还有其他两个可选参数。
参数
设置为 −
参数 | 描述 |
---|---|
to | 必需。指定电子邮件的收件人 |
subject | 必需。指定电子邮件的主题。此参数不能包含任何换行符 |
message | 必需。定义要发送的消息。每行应用 LF (\n) 分隔。行数不应超过 70 个字符 |
headers | 可选。指定其他标题,如 From、Cc 和 Bcc。其他标头应使用 CRLF (\r\n) 分隔 |
parameters | 可选。指定发送邮件程序的附加参数 |
可以将多个收件人指定为逗号分隔列表中 mail() 函数的第一个参数。
发送 HTML 电子邮件
当您使用 PHP 发送短信时,所有内容都将被视为简单文本。即使您将在文本消息中包含 HTML 标签,它也会显示为简单文本,并且 HTML 标签不会根据 HTML 语法进行格式化。
但是 PHP 提供了将 HTML 消息作为实际 HTML 消息发送的选项。
发送电子邮件时,您可以指定 Mime 版本、内容类型和字符集以发送 HTML 电子邮件。
例子
以下示例显示了如何将 HTML 电子邮件消息发送到“xyz@qikepu.com”,并将其复制到“afgh@qikepu.com”。您可以以这样一种方式编写此程序,使其应接收来自用户的所有内容,然后应发送电子邮件。
它应该接收来自用户的所有内容,然后应该发送电子邮件。
它将产生以下输出 -
sh: 1: /usr/sbin/sendmail: not found
从 Localhost 发送电子邮件
上述调用 PHP mail 的方法可能不适用于您的本地主机。在这种情况下,还有发送电子邮件的替代解决方案。您可以使用 PHPMailer 通过 SMTP 从 localhost 发送电子邮件。
PHPMailer 是一个开源库,用于连接 SMTP 以发送电子邮件。您可以从 PEAR 或 Composer 存储库下载它,也可以从 https://github.com/PHPMailer/PHPMailer 下载它。从此处下载 ZIP 文件,将 PHPMailer 文件夹的内容复制到 PHP 配置中指定的 include_path 目录之一,然后手动加载每个类文件。
例子
使用以下 PHP 脚本通过 PHPMailer 库发送电子邮件 -
Phpmailer.php
使用以下 HTML 表单编写邮件消息。表单将提交到上述 phpmail.php 脚本
Email.html
通过电子邮件发送附件
要发送包含混合内容的电子邮件,您应该将 Content-type 标头设置为 multipart/mixed。然后,可以在边界内指定文本和附件部分。
边界以两个连字符开头,后跟一个唯一编号,该编号不能显示在电子邮件的消息部分中。PHP 函数 md5 用于创建一个 32 位十六进制数以创建唯一数字。表示电子邮件最后一部分的最后一个边界也必须以两个连字符结尾。
请看下面的例子 -
它将产生以下输出 -
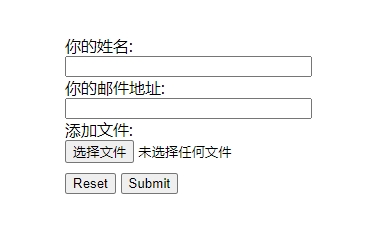