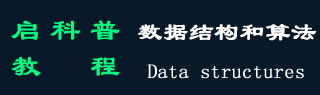
- Python 数据结构和算法教程
- Python - 数据结构教程
- Python - 数据结构简介
- Python - 数据结构环境
- Python - 二维数组的数据结构
- Python - 矩阵的数据结构
- Python - 地图的数据结构
- Python - 链表的数据结构
- Python - 堆栈的数据结构
- Python - 队列的数据结构
- Python - 取消排队
- Python - 高级链表
- Python - 哈希表的数据结构
- Python - 二叉树
- Python - 二叉搜索树
- Python - 堆数据结构
- Python - 图形数据结构
- Python - 算法设计
- Python - 分治算法
- Python - 回溯
- Python - 排序算法
- Python - 搜索算法
- Python - 图形算法
- Python - 算法分析
- Python - 算法类型
- Python - 算法类
- Python - 摊销分析
- Python - 算法理由
Python - 哈希表的数据结构
哈希表是一种数据结构,其中数据元素的地址或索引值是从哈希函数生成的。这使得访问数据的速度更快,因为索引值的行为类似于数据值的键。换句话说,哈希表存储键值对,但键是通过哈希函数生成的。
因此,数据元素的搜索和插入功能会变得更快,因为键值本身成为存储数据的数组的索引。
在 Python 中,Dictionary 数据类型表示哈希表的实现。字典中的 Key 满足以下要求。
- 字典的键是可哈希的,即由哈希函数生成,该函数为提供给哈希函数的每个唯一值生成唯一结果。
- 字典中数据元素的顺序不是固定的。
因此,我们看到使用字典数据类型实现哈希表,如下所示。
访问 Dictionary 中的值
要访问字典元素,您可以使用熟悉的方括号和 key 来获取其值。
例
# Declare a dictionary
dict = {'Name': 'Zara', 'Age': 7, 'Class': 'First'}
# Accessing the dictionary with its key
print ("dict['Name']: ", dict['Name'])
print ("dict['Age']: ", dict['Age'])
输出
执行上述代码时,它会产生以下结果 -
dict['Name']: Zara
dict['Age']: 7
dict['Age']: 7
更新词典
您可以通过添加新条目或键值对、修改现有条目或删除现有条目来更新字典,如下面的简单示例所示 -
例
# Declare a dictionary
dict = {'Name': 'Zara', 'Age': 7, 'Class': 'First'}
dict['Age'] = 8; # update existing entry
dict['School'] = "DPS School"; # Add new entry
print ("dict['Age']: ", dict['Age'])
print ("dict['School']: ", dict['School'])
输出
执行上述代码时,它会产生以下结果 -
dict['Age']: 8
dict['School']: DPS School
dict['School']: DPS School
删除字典元素
您可以删除单个词典元素或清除词典的全部内容。您还可以在单个操作中删除整个词典。要显式删除整个字典,只需使用 del 语句。
例
dict = {'Name': 'Zara', 'Age': 7, 'Class': 'First'}
del dict['Name']; # remove entry with key 'Name'
dict.clear(); # remove all entries in dict
del dict ; # delete entire dictionary
print ("dict['Age']: ", dict['Age'])
print ("dict['School']: ", dict['School'])
输出
这将产生以下结果。请注意,引发异常是因为 after del dict 字典不再存在。
dict['Age']: dict['Age']
dict['School']: dict['School']
dict['School']: dict['School']